Databases | Tasks for Practical Class 5
Creating queries using the GROUP BY
and ORDER BY
clauses. Creating queries with the HAVING
clause.
Variant 1: Online Bookstore Sales
Schema:
CREATE TABLE orders (
order_id INTEGER PRIMARY KEY,
customer_id INTEGER,
order_date DATE,
book_title VARCHAR(255),
genre VARCHAR(255),
quantity INTEGER,
price_per_unit NUMERIC(10, 2)
);
INSERT INTO orders (order_id, customer_id, order_date, book_title, genre, quantity, price_per_unit) VALUES
(1, 101, '2024-01-15', 'The Mystery of the Lost Key', 'Mystery', 2, 15.99),
(2, 102, '2024-01-20', 'Space Odyssey 2045', 'Science Fiction', 1, 24.99),
(3, 101, '2024-02-05', 'Cooking with Passion', 'Cookbook', 3, 12.50),
(4, 103, '2024-02-10', 'The Mystery of the Lost Key', 'Mystery', 1, 15.99),
(5, 102, '2024-02-15', 'Learn Python in 30 Days', 'Programming', 2, 19.99),
(6, 104, '2024-02-20', 'Space Odyssey 2045', 'Science Fiction', 1, 24.99),
(7, 101, '2024-03-01', 'The History of Rome', 'History', 1, 9.99),
(8, 103, '2024-03-05', 'Cooking with Passion', 'Cookbook', 2, 12.50),
(9, 105, '2024-03-10', 'Quantum Physics for Beginners', 'Science', 1, 29.99),
(10, 104, '2024-03-12', 'Business Strategy Masterclass', 'Business', 1, 39.99);
Tasks:
- List all the unique genres in the
orders
table, ordered alphabetically. - List all book titles that have the word “the” (case-insensitive) in them, ordered by title.
- List the genres from the
orders
table which have titles containing the word “of”, ordered alphabetically. - List all book titles, ordered by book title DESC.
- Find genres containing letter ‘e’, considering only books with
price_per_unit
higher than 16.00. Order result by genre. - List all distinct book titles, ordered by the length of the title (shortest to longest).
- List all genres that end with the letter “e”, ordered alphabetically.
- Show all book titles that contain the letter “a” and the letter “s”, ordered by title.
- List genres where the book titles contain digits, ordered alphabetically.
- List book titles that start with ‘The’ and contain ‘Mystery’, ordered by
price_per_unit
DESC.
Variant 2: University Course Enrollment
Schema:
CREATE TABLE courses (
course_id INTEGER PRIMARY KEY,
course_name VARCHAR(255),
department VARCHAR(255),
credits INTEGER,
grade CHAR(1)
);
INSERT INTO courses (course_id, course_name, department, credits, grade) VALUES
(101, 'Introduction to Programming', 'Computer Science', 3, 'A'),
(102, 'Calculus I', 'Mathematics', 4, 'B'),
(103, 'World History', 'History', 3, 'A'),
(104, 'Linear Algebra', 'Mathematics', 3, 'C'),
(105, 'Data Structures and Algorithms', 'Computer Science', 4, 'A'),
(106, 'Probability and Statistics', 'Statistics', 3, 'B'),
(107, 'Introduction to Programming', 'Computer Science', 3, 'B');
Tasks:
- List all the unique departments in the
courses
table, ordered alphabetically. - List the course names that contain the word “and”, ordered by course name.
- List the departments that offer courses with names containing “Pro”, ordered alphabetically.
- List the course names with a grade of ‘A’, ordering by name DESC.
- Find course names that end with ‘s’, considering only courses with credits equal to 3. Order result alphabetically.
- List all distinct department names, ordered by the length of the department name (longest to shortest).
- List departments that start with the letter “C”, ordered alphabetically.
- Show course names containing the letter ‘i’ at least twice, ordered by course name.
- List departments where course names include word ‘to’, ordered alphabetically.
- Find course names starting with ‘I’ and having grade ‘B’, order by
credits
.
Variant 3: Employee Project Management
Schema:
CREATE TABLE projects (
project_id INTEGER PRIMARY KEY,
project_name VARCHAR(255),
department VARCHAR(255),
start_date DATE,
end_date DATE,
budget NUMERIC(12, 2)
);
INSERT INTO projects (project_id, project_name, department, start_date, end_date, budget) VALUES
(101, 'Website Redesign', 'IT', '2024-01-15', '2024-03-15', 50000.00),
(102, 'New Marketing Campaign', 'Marketing', '2024-02-01', '2024-04-30', 75000.00),
(103, 'Database Migration', 'IT', '2024-03-01', '2024-05-31', 100000.00),
(104, 'Financial Reporting System', 'Finance', '2024-01-01', '2024-06-30', 120000.00),
(105, 'New Database Design', 'IT', '2024-04-01', '2024-06-01', 90000.00);
Tasks:
- List all unique departments in the
projects
table, ordered in descending alphabetical order. - List the project names that start with “New”, ordered by project name.
- List departments that have projects with names containing “Data”.
- List project names, ordering by project name.
- List the project names that have a budget greater than 60000, and that end with ‘n’, ordering the result alphabetically.
- List all distinct department names, ordered by the length of the department name (shortest to longest).
- List project names that contain the word “New” or the word “System”, ordered by project name.
- Show departments having projects which names contain letter ‘b’ and letter ‘e’, ordered alphabetically.
- List departments where project names contain space, ordered alphabetically.
- List project names where start date is later than ‘2024-02-01’ and name contains ‘Data’, ordering by
budget
DESC.
Variant 4: Music Streaming Service
Schema:
CREATE TABLE songs (
song_id INTEGER PRIMARY KEY,
song_name VARCHAR(255),
artist_name VARCHAR(255),
genre VARCHAR(255),
album_name VARCHAR(255),
duration_seconds INTEGER
);
INSERT INTO songs (song_id, song_name, artist_name, genre, album_name, duration_seconds) VALUES
(1, 'Come Together', 'The Beatles', 'Rock', 'Abbey Road', 259),
(2, 'Something', 'The Beatles', 'Rock', 'Abbey Road', 182),
(3, 'So What', 'Miles Davis', 'Jazz', 'Kind of Blue', 556),
(4, 'Blue in Green', 'Miles Davis', 'Jazz', 'Kind of Blue', 337),
(5, 'Stairway to Heaven', 'Led Zeppelin', 'Rock', 'Led Zeppelin IV', 482),
(6, 'Black Dog', 'Led Zeppelin', 'Rock', 'Led Zeppelin IV', 296),
(7, 'Blank Space', 'Taylor Swift', 'Pop', '1989', 231),
(8, 'Shake It Off', 'Taylor Swift', 'Pop', '1989', 219),
(9, 'Alright', 'Kendrick Lamar', 'Hip Hop', 'To Pimp a Butterfly', 219),
(10, 'King Kunta', 'Kendrick Lamar', 'Hip Hop', 'To Pimp a Butterfly', 234);
Tasks:
- List all the unique genres from the
songs
table, ordered alphabetically. - List all album names, ordered by album name in descending order.
- List the genres of songs with names containing “Blue”.
- List song names that have artist name ‘The Beatles’, ordering by song name DESC.
- Find the album names containing the word “of”, ordered alphabetically.
- List all distinct artist names, ordered by the length of the artist name (shortest to longest).
- List genres that contain the letter “o” and the letter “p”, ordered alphabetically.
- Show song names which contain character ‘k’ at least twice, ordered by the song name.
- List artist names where song name contains ‘So’, ordered alphabetically.
- List song names which have
duration_seconds
greater than 250 and contain word ‘Black’, order byduration_seconds
.
Variant 5: Library Management System
Schema:
CREATE TABLE books (
book_id INTEGER PRIMARY KEY,
title VARCHAR(255),
author VARCHAR(255),
genre VARCHAR(255),
publication_year INTEGER,
loaned BOOLEAN
);
INSERT INTO books (book_id, title, author, genre, publication_year, loaned) VALUES
(1, 'The Lord of the Rings', 'J.R.R. Tolkien', 'Fantasy', 1954, TRUE),
(2, 'Pride and Prejudice', 'Jane Austen', 'Romance', 1813, TRUE),
(3, '1984', 'George Orwell', 'Dystopian', 1949, TRUE),
(4, 'To Kill a Mockingbird', 'Harper Lee', 'Fiction', 1960, FALSE),
(5, 'The Catcher in the Rye', 'J.D. Salinger', 'Fiction', 1951, TRUE);
Tasks:
- List unique genres from the
books
table in descending alphabetical order. - List the book titles published before 1950, ordered by publication year.
- List the genres of books with titles containing the word “the”, ordered alphabetically.
- List author names, ordering by author name.
- List all books titles that are currently loaned (
loaned
is TRUE), and title ends with ‘e’, ordering by title. - List all distinct author names, ordered by the length of the author name (longest to shortest).
- List genres that start with the letter “F” or the letter “R”, ordered alphabetically.
- Show book titles that contain the letter “a” and are currently loaned, ordered by title.
- List genres of books, which titles contain ‘and’, ordered by genre.
- List titles of books published after 1950 and with author name starting with ‘J’, order by publication year.
Variant 6: E-commerce Product Catalog
Schema:
CREATE TABLE products (
product_id INTEGER PRIMARY KEY,
product_name VARCHAR(255),
category_name VARCHAR(255),
price NUMERIC(10, 2),
stock_quantity INTEGER
);
INSERT INTO products (product_id, product_name, category_name, price, stock_quantity) VALUES
(1, 'Laptop', 'Electronics', 1200.00, 50),
(2, 'T-Shirt', 'Clothing', 25.00, 200),
(3, 'The Hitchhiker''s Guide to the Galaxy', 'Books', 15.00, 100),
(4, 'Coffee Maker', 'Home & Kitchen', 75.00, 75),
(5, 'Smartphone', 'Electronics', 800.00, 100),
(6, 'Jeans', 'Clothing', 50.00, 150),
(7, 'A Brief History of Time', 'Books', 20.00, 80),
(8, 'Blender', 'Home & Kitchen', 100.00, 60);
Tasks:
- List all unique category names from the
products
table in descending alphabetical order. - List product names that have a price greater than $50, ordering by product name.
- List the category names that have products with names containing “of”.
- List product names from category ‘Electronics’, ordering by product name DESC.
- Find product names that have price less than 1000 and containing the word ‘the’, ordered by name.
- List all distinct category names, ordered by the length of the category name (shortest to longest).
- List category names containing ‘c’ and ‘n’ ordered alphabetically.
- List all product names that start with the letter ‘B’, ordered by product name.
- List category names where product names contain character ‘p’ at least twice, ordered by category name.
- Find product names with
stock_quantity
higher than 80 and containing ‘er’, ordered byprice
.
Submission Instructions
- Create a new Google Document. Example
- Submit Your Google Doc Link: Google Sheet
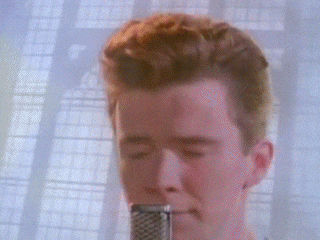