Databases | Tasks for Practical Class 3
Creating, Modifying, and Deleting Tables in SQL
Variant 1: Online Bookstore Database
Variant 1: Online Bookstore Database
Scenario: You are tasked with setting up a database for an online bookstore. This database will initially manage books and their authors.
Table Schemas:
books
table:book_id
: Unique identifier for each book (auto-incrementing integer, primary key).title
: Title of the book (text, not null, maximum length 100 characters).isbn
: International Standard Book Number (text, unique, maximum length 20 characters).publication_year
: Year of publication (integer).genre
: Genre of the book (text, maximum length 50 characters).
authors
table:author_id
: Unique identifier for each author (auto-incrementing integer, primary key).author_name
: Name of the author (text, not null, maximum length 100 characters).nationality
: Nationality of the author (text, maximum length 50 characters).birth_date
: Date of birth of the author (date).
Tasks:
- Create the
books
andauthors
tables in your database using theCREATE TABLE
statement. - Add two new columns to the
books
table:price
(decimal) andstock_quantity
(integer, default value 0). - Modify the
genre
column in thebooks
table to have a maximum length of 75 characters and change the data type ofpublication_year
toSMALLINT
. - Rename the
author_name
column in theauthors
table toname
and rename thenationality
column tocountry
. - Drop the
birth_date
column from theauthors
table and thegenre
column from thebooks
table. - Delete the
authors
table from the database using theDROP TABLE
statement.
Variant 2: Event Management Database
Variant 2: Event Management Database
Scenario: You are building a database to manage events and venues for an event planning company.
Table Schemas:
events
table:event_id
: Unique identifier for each event (auto-incrementing integer, primary key).event_name
: Name of the event (text, not null, maximum length 100 characters).event_date
: Date of the event (date, not null).start_time
: Time the event starts (time).category
: Category of the event (text, maximum length 50 characters).
venues
table:venue_id
: Unique identifier for each venue (auto-incrementing integer, primary key).venue_name
: Name of the venue (text, not null, maximum length 100 characters).address
: Address of the venue (text, maximum length 200 characters).capacity
: Maximum capacity of the venue (integer).venue_type
: Type of venue (e.g., ‘hall’, ‘stadium’, ‘park’) (text, maximum length 50 characters).
Tasks:
- Create the
events
andvenues
tables in your database. - Add two new columns to the
events
table:description
(text) andis_cancelled
(boolean, default false). - Modify the
category
column in theevents
table to beNOT NULL
and change the data type ofcapacity
in thevenues
table toBIGINT
. - Rename the
event_name
column in theevents
table totitle
and rename thevenue_name
column in thevenues
table toname
. - Drop the
start_time
column from theevents
table and thevenue_type
column from thevenues
table. - Delete the
venues
table from the database.
Variant 3: Music Library Database
Variant 3: Music Library Database
Scenario: You are creating a database to manage a personal music library, tracking songs and artists.
Table Schemas:
songs
table:song_id
: Unique identifier for each song (auto-incrementing integer, primary key).song_title
: Title of the song (text, not null, maximum length 100 characters).duration_seconds
: Duration of the song in seconds (integer).release_year
: Year the song was released (integer).genre
: Genre of the song (text, maximum length 50 characters).
artists
table:artist_id
: Unique identifier for each artist (auto-incrementing integer, primary key).artist_name
: Name of the artist (text, not null, maximum length 100 characters).origin_country
: Country of origin of the artist (text, maximum length 50 characters).formation_year
: Year the artist was formed (integer).
Tasks:
- Create the
songs
andartists
tables in your database. - Add two new columns to the
songs
table:album_name
(text, maximum length 100 characters) andplay_count
(integer, default 0). - Modify the
genre
column in thesongs
table to have a maximum length of 75 characters and change the data type ofduration_seconds
toREAL
. - Rename the
song_title
column in thesongs
table totitle
and rename theartist_name
column in theartists
table toname
. - Drop the
release_year
column from thesongs
table and theformation_year
column from theartists
table. - Delete the
artists
table from the database.
Variant 4: Simple Blogging Platform Database
Variant 4: Simple Blogging Platform Database
Scenario: You are designing a database for a simple blogging platform to manage blog posts and categories.
Table Schemas:
posts
table:post_id
: Unique identifier for each post (auto-incrementing integer, primary key).post_title
: Title of the blog post (text, not null, maximum length 200 characters).content
: Content of the blog post (text).publication_date
: Date the post was published (date, default current date).is_published
: Status of publication (boolean, default false).
categories
table:category_id
: Unique identifier for each category (auto-incrementing integer, primary key).category_name
: Name of the category (text, not null, unique, maximum length 50 characters).description
: Description of the category (text, maximum length 200 characters).created_at
: Timestamp when the category was created (timestamp with time zone, default current timestamp).
Tasks:
- Create the
posts
andcategories
tables in your database. - Add two new columns to the
posts
table:author_name
(text, maximum length 100 characters) andview_count
(integer, default 0). - Modify the
post_title
column in theposts
table to have a maximum length of 255 characters and change the data type ofpublication_date
toTIMESTAMP
. - Rename the
post_title
column in theposts
table totitle
and rename thecategory_name
column in thecategories
table toname
. - Drop the
is_published
column from theposts
table and thedescription
column from thecategories
table. - Delete the
categories
table from the database.
Variant 5: Recipe Database
Variant 5: Recipe Database
Scenario: You are creating a database to store recipes and their ingredients for a recipe application.
Table Schemas:
recipes
table:recipe_id
: Unique identifier for each recipe (auto-incrementing integer, primary key).recipe_name
: Name of the recipe (text, not null, maximum length 100 characters).instructions
: Cooking instructions (text).cuisine_type
: Type of cuisine (e.g., ‘Italian’, ‘Mexican’, ‘Indian’) (text, maximum length 50 characters).preparation_time_minutes
: Preparation time in minutes (integer).
ingredients
table:ingredient_id
: Unique identifier for each ingredient (auto-incrementing integer, primary key).ingredient_name
: Name of the ingredient (text, not null, unique, maximum length 100 characters).is_vegetarian
: Indicates if the ingredient is vegetarian (boolean, default true).is_vegan
: Indicates if the ingredient is vegan (boolean, default false).
Tasks:
- Create the
recipes
andingredients
tables in your database. - Add two new columns to the
recipes
table:servings
(integer, default 1) andrating
(numeric, precision 2, scale 1, e.g., 4.5). - Modify the
cuisine_type
column in therecipes
table to have a maximum length of 75 characters and change the data type ofpreparation_time_minutes
toSMALLINT
. - Rename the
recipe_name
column in therecipes
table toname
and rename theingredient_name
column in theingredients
table toname
. - Drop the
instructions
column from therecipes
table and theis_vegan
column from theingredients
table. - Delete the
ingredients
table from the database.
Variant 6: Simple Task Management Database
Variant 6: Simple Task Management Database
Scenario: You are building a database for a simple task management application to manage tasks and projects.
Table Schemas:
tasks
table:task_id
: Unique identifier for each task (auto-incrementing integer, primary key).task_name
: Name of the task (text, not null, maximum length 100 characters).description
: Description of the task (text).due_date
: Date the task is due (date).status
: Status of the task (e.g., ‘To Do’, ‘In Progress’, ‘Completed’) (text, maximum length 50 characters, default ‘To Do’).
projects
table:project_id
: Unique identifier for each project (auto-incrementing integer, primary key).project_name
: Name of the project (text, not null, maximum length 100 characters).start_date
: Date the project started (date).end_date
: Date the project is expected to end (date).priority
: Priority of the project (e.g., ‘High’, ‘Medium’, ‘Low’) (text, maximum length 50 characters, default ‘Medium’).
Tasks:
- Create the
tasks
andprojects
tables in your database. - Add two new columns to the
tasks
table:created_at
(timestamp with time zone, default current timestamp) andis_urgent
(boolean, default false). - Modify the
status
column in thetasks
table to have a maximum length of 75 characters and change the data type ofdue_date
toTIMESTAMP
. - Rename the
task_name
column in thetasks
table totitle
and rename theproject_name
column in theprojects
table toname
. - Drop the
description
column from thetasks
table and theend_date
column from theprojects
table. - Delete the
projects
table from the database.
Submission Instructions
1. Perform the Tasks:
- For your selected variant, complete all the tasks outlined. This involves writing and executing SQL commands in pgAdmin 4 using PostgreSQL.
2. Document Your Work in a Google Doc:
- Create a new Google Document. example
- At the top of the document, clearly write:
- Your Name:
- Variant Number: (e.g., Variant 1: Online Bookstore Database)
- For each task within the variant, document the following:
- Task Number: (e.g., Task 1, Task 2, Task 3, etc.)
- SQL Command: Copy and paste the exact SQL command you executed in pgAdmin 4 for that task.
- Confirmation/Screenshot: Include a screenshot from pgAdmin 4 (showing the table created in the Object Browser) to visually confirm the successful execution of your command.
3. Submit Your Google Doc Link:
- Once you have completed documenting all tasks for your chosen variant in your Google Doc, ensure the document is shared so that your teacher can access it. Important: Set the sharing permissions to “Anyone with the link can Comment”.
- Go to the Google Sheet provided by your teacher for assignment submissions. Google Sheet
- Locate the column corresponding to your name.
- In the appropriate cell for this Database Assignment, paste the shareable link to your Google Doc.
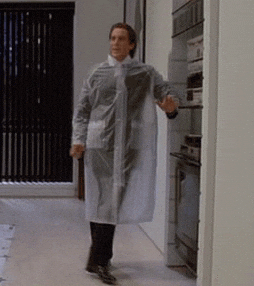